Kotlin Coroutines In-Depth
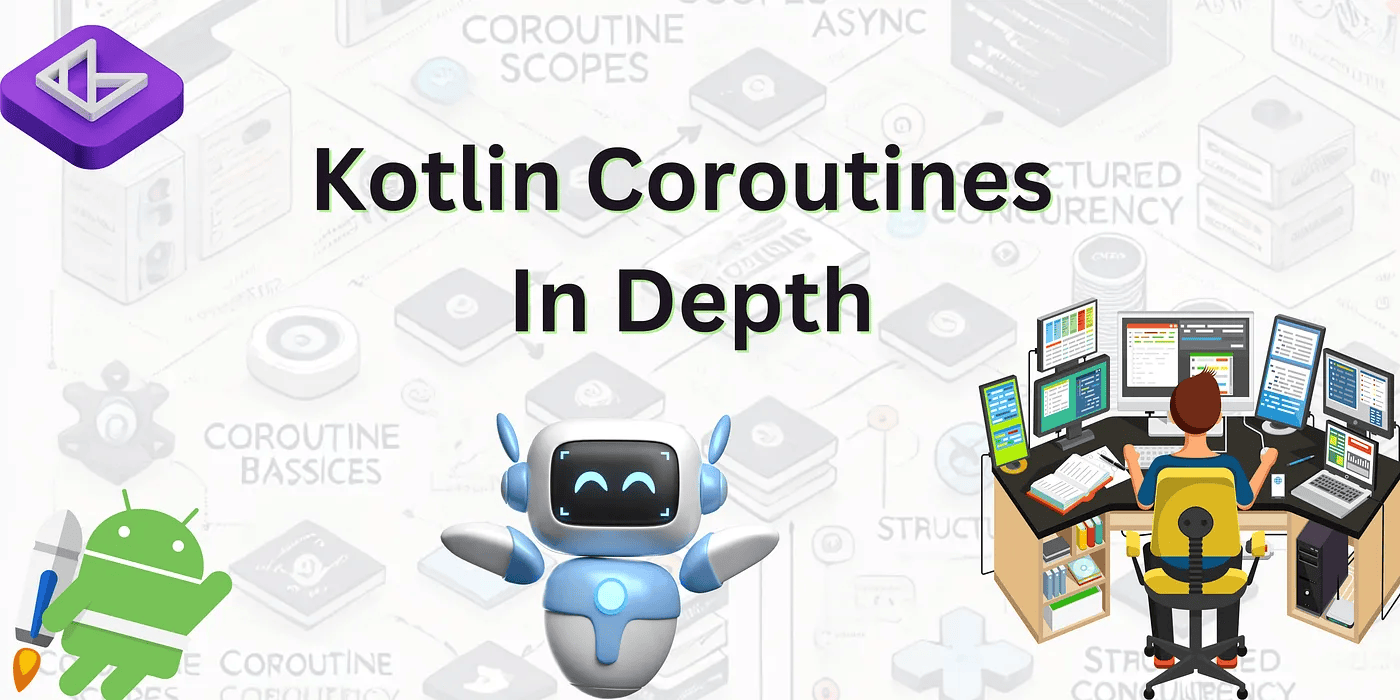
Asynchronous programming is essential in modern mobile development to keep apps responsive and avoid blocking the main thread. Kotlin Coroutines provide a powerful yet straightforward way to handle asynchronous tasks without the complexity of callbacks or traditional threading.
In this blog, we’ll explore what Kotlin Coroutines are, how they work, and how you can use them to handle asynchronous tasks efficiently. Plus, we’ll walk through examples, including real-life use cases, to show how coroutines make code cleaner and more manageable.
What Are Coroutines?
Coroutines are like lightweight threads. They let us run long or complex tasks in the background (such as network calls, database operations, or complex calculations) without freezing the main thread or making the app unresponsive. Coroutines can be paused and resumed, freeing up system resources when they’re not actively doing something.
Coroutines are a feature in Kotlin that helps you write asynchronous code in a sequential, readable style. They allow you to run tasks concurrently without blocking the main thread. Coroutines work with lightweight threads, meaning you can run multiple coroutines at once without heavy resource consumption.
Why Use Coroutines?
In Android, if we run long tasks (like fetching data from the internet) on the main thread, the app will “freeze,” causing a poor user experience. Coroutines solve this problem by allowing us to keep these tasks off the main thread while still keeping the code simple and readable.
Key Concepts in Kotlin Coroutines
- Coroutine Scope: A scope defines the lifetime of a coroutine, which controls when it starts and when it gets canceled.
- Dispatcher: Tells the coroutine which thread to run on. For example:
Dispatchers.Main
: Runs on the main thread (used for UI tasks).Dispatchers.IO
: Runs on a background thread for I/O tasks like network or database work.Dispatchers.Default
: Used for CPU-intensive tasks.- Suspend Functions: Functions marked with the
suspend
keyword, allowing them to pause and resume, making them ideal for long-running tasks like network calls or database operations. - Job and Deferred: A
Job
represents a cancellable coroutine, whileDeferred
is a subclass ofJob
that returns a result, similar toFuture
in other languages. - Structured Concurrency: Coroutines in Kotlin follow structured concurrency, meaning they automatically manage cancellation and exception handling within a defined scope.
Real-Life Scenario: Fetching Data from an API
One of the most common uses of coroutines in Android is fetching data from a remote server. Let’s start by setting up a coroutine to make a network call.
import kotlinx.coroutines.* // Simulating network data fetch with suspend function suspend fun fetchWeatherData(city: String): String { delay(2000) // Simulate network delay return "Sunny, 24°C in $city" } // Main function to run the coroutine fun main() = runBlocking { println("Fetching weather data...") val weather = fetchWeatherData("New York") println("Weather data: $weather") }
In this example:
runBlocking
creates a coroutine scope and blocks the main thread until the coroutine inside completes. This is mainly for testing and should not be used in production UI code.delay
simulates a network delay, allowing the coroutine to pause without blocking the thread.
Output:
Fetching weather data... Weather data: Sunny, 24°C in New York
Understanding launch
and async
When working with coroutines, you’ll use either launch
or async
to start coroutines:
1. Launch coroutine
- Usage:
launch
is primarily used when we want to perform a task that doesn’t need to return a result, like updating a UI or performing a side effect. - Return Type:
launch
does not return a result; instead, it returns aJob
, which represents the coroutine and can be used to manage its lifecycle (such as canceling it).
Example: Loading data in the background without expecting a return value.
fun performTask() { CoroutineScope(Dispatchers.IO).launch { // Perform a long-running task delay(1000) println("Task complete") } }
2. async
Coroutine
- Usage:
async
is used when we want to perform a task that returns a result, often in parallel with other tasks. - Return Type:
async
returns aDeferred
object, which represents a promise of future data. We can call.await()
on theDeferred
to get the result when the task completes.
Example: Fetching data from multiple sources at the same time and combining results.
fun fetchResults() = CoroutineScope(Dispatchers.IO).launch { val deferredResult = async { getDataFromServer() } val result = deferredResult.await() println("Received result: $result") } suspend fun getDataFromServer(): String { delay(1000) // Simulates a network call return "Data from server" }
Example: Launching Multiple Tasks in Parallel
Suppose you’re building a dashboard that needs to fetch user data and notification count at the same time:
suspend fun fetchUserData(): String { delay(1000) // Simulate API call return "User: Jane Doe" } suspend fun fetchNotificationCount(): Int { delay(500) // Simulate API call return 5 } fun main() = runBlocking { println("Fetching dashboard data...") val userDeferred = async { fetchUserData() } val notificationsDeferred = async { fetchNotificationCount() } // Wait for both results to complete val userData = userDeferred.await() val notifications = notificationsDeferred.await() println("Dashboard Data: $userData, Notifications: $notifications") }
This example runs both fetchUserData()
and fetchNotificationCount()
in parallel using async
. By calling await()
on each, we ensure that we get the result once both tasks complete.
Coroutine Scopes in Android
In Android, coroutines are typically launched in a specific CoroutineScope
to prevent memory leaks and manage the coroutine lifecycle. Common coroutine scopes in Android include:
viewModelScope
: Used within ViewModels to handle coroutines tied to the ViewModel’s lifecycle. Automatically cancels the coroutine if the ViewModel is cleared.lifecycleScope
: Used within activities and fragments. It automatically cancels coroutines when the activity or fragment is destroyed.GlobalScope
: A global coroutine scope that lives throughout the application’s lifecycle. Use it sparingly, as it does not automatically cancel coroutines.
Real-Life Scenario: Loading Data on the Main Thread with lifecycleScope
Imagine a screen in your app where you need to fetch a user profile from an API and display it. You want the data-fetching to happen in the background to keep the UI responsive.
class ProfileFragment : Fragment() { override fun onViewCreated(view: View, savedInstanceState: Bundle?) { super.onViewCreated(view, savedInstanceState) // Using lifecycleScope to manage coroutine within Fragment's lifecycle lifecycleScope.launch(Dispatchers.IO) { val profileData = fetchUserProfile() withContext(Dispatchers.Main) { // Update UI on the main thread textViewProfile.text = profileData } } } private suspend fun fetchUserProfile(): String { delay(2000) // Simulate API delay return "Jane Doe, Software Engineer" } }
In this example:
- We use
lifecycleScope
to ensure the coroutine is canceled if the fragment is destroyed. Dispatchers.IO
is used for network calls to avoid blocking the main thread.withContext(Dispatchers.Main)
is used to update the UI on the main thread after fetching data.
Error Handling with Coroutines
Error handling in coroutines is essential. Kotlin provides try-catch
blocks to handle exceptions in coroutines, but there are also structured approaches like supervisorScope
.
Example: Handling Network Errors with try-catch
suspend fun fetchData(): String { delay(1000) throw Exception("Network Error") } fun main() = runBlocking { try { val data = fetchData() println("Data fetched: $data") } catch (e: Exception) { println("Error occurred: ${e.message}") } }
Output:
Error occurred: Network Error
This try-catch
block catches any exceptions that occur during the coroutine’s execution.
Why Use Coroutines? Advantages for Real-Life Applications
- Simplifies Asynchronous Code: Coroutines eliminate callback hell, making asynchronous code more readable and maintainable.
- Improves Performance: Coroutines are lightweight and use fewer resources than traditional threads, allowing you to handle multiple tasks in parallel efficiently.
- Scoped Lifecycle: In Android, coroutine scopes (like
viewModelScope
) help prevent memory leaks by automatically canceling coroutines when they’re no longer needed.
Why Kotlin Coroutines Are Great
- Readable Code: Coroutines make asynchronous code look synchronous and straightforward.
- Lightweight: Unlike traditional threads, coroutines are cheap and don’t consume much memory.
- Built-in cancellation support: Cancellation is generated automatically through the running coroutine hierarchy.
- Fewer memory leaks: It uses structured concurrency to run operations within a scope.
- Structured Concurrency: Coroutine scopes and structured concurrency allow you to control and manage coroutine lifecycles, ensuring they’re automatically canceled when no longer needed.
- Jetpack integration: Many Jetpack libraries include extensions that provide full coroutines support. Some libraries also provide their own coroutine scope that one can use for structured concurrency.
Kotlin Coroutines vs Threads
- Lightweight: Coroutines are far lighter on resources. You can run thousands in a single thread, unlike threads, which are resource-intensive.
- Non-blocking: Coroutines suspend without blocking the thread, freeing it for other tasks. Threads block while waiting, limiting responsiveness.
- Efficiency: Coroutines switch context faster since it’s managed by the library, not the OS. Thread context switching is heavy and slower.
- Error Handling: Coroutines provide structured error handling with easy cancellation, making it simpler to manage errors and cleanup. Threads have limited mechanisms for managing errors and cancellations.
- Ease of Use: Coroutines integrate well with Kotlin, reducing the complexity of asynchronous code, especially on Android. Managing multiple threads is more cumbersome.
Summary
Kotlin Coroutines bring the power of asynchronous programming to Android with simplicity, flexibility, and performance. Whether fetching data from an API, handling complex background tasks, or updating the UI in real-time, coroutines provide a cleaner and more efficient way to manage concurrent tasks.